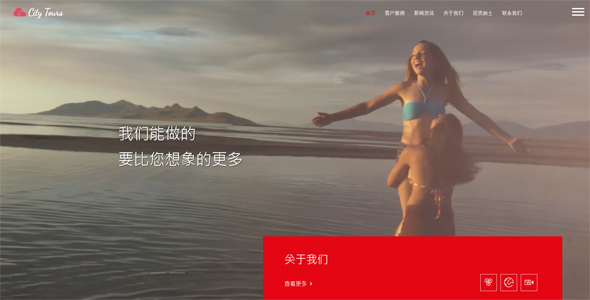
Avatars 是一款可以动态生成用户头像 js 插件。Avatars 可以在浏览器或 node.js 环境中,动态生成各种漂亮的、基于 svg 的用户头像。
$ npm install @dicebear/avatars --save
然后选择以下的头像类型进行安装。
# male
$ npm install @dicebear/avatars-male-sprites --save
# female
$ npm install @dicebear/avatars-female-sprites --save
# identicon
$ npm install @dicebear/avatars-identicon-sprites --save
# initials
$ npm install @dicebear/avatars-initials-sprites --save
# bottts
$ npm install @dicebear/avatars-bottts-sprites --save
# gridy
$ npm install @dicebear/avatars-gridy-sprites --save
# avataaars
$ npm install @dicebear/avatars-avataaars-sprites --save
# jdenticon
$ npm install @dicebear/avatars-jdenticon-sprites --save
import Avatars from '@dicebear/avatars';
import SpriteCollection from '@dicebear/avatars-male-sprites';
let avatars = new Avatars(SpriteCollection);
let svg = avatars.create('custom-seed');
例如,要创建一个男生头像:
let options = {};
let avatars = new Avatars(sprites(options));
let svg = avatars.create('custom-seed');
create()中的 custom-seed 是一个随机任意的字符串,随着字符串的不同,生成的头像也不相同。
male-sprites 和 female-sprites 的配置参数:
let options = {
mood: ['happy', 'sad', 'surprised']
};
identicon-sprites 的配置参数:
let options = {
// Distance to the edge of the image
padding: 0,
// Background color
background: #FFF
};
bottts-sprites 的配置参数:
let options = {
// Possible values: amber, blue, blueGrey, brown, cyan, deepOrange, deepPurple, agreenmber, grey, indigo, lightBlue, lightGreen, lime, orange, pink, purple, red, teal, yellow
colors: [],
// Possible values: 50, 100, 200, 300, 400, 500, 600, 700, 800, 900
primaryColorLevel: 600,
// Possible values: 50, 100, 200, 300, 400, 500, 600, 700, 800, 900
secondaryColorLevel: 400,
// in percent
colorful: 100,
mouthChance: 100,
sidesChance: 100,
textureChance: 50,
topChange: 100,
};
avataaars-sprites 的配置参数:
let options = {
// transparent, circle
style: 'transparent'
// include or exclude passed options.
mode: 'include',
// Possible values: longHair, shortHair, eyepatch, hat, hijab, turban
top: [],
// in percent
topChance: 100,
// Possible values: black, blue, gray, heather, pastel, pink, red, white
hatColor: [],
// Possible values: auburn, black, blonde, brown, pastel, platinum, red, gray
hairColor: [],
// Possible values: kurt, prescription01, prescription02, round, sunglasses, wayfarers
accessories: [],
// in percent
accessoriesChance: 10,
// Possible values: medium, light, magestic, fancy, magnum
facialHair: null,
// in percent
facialHairChance: 10,
// Possible values: auburn, black, blonde, brown, platinum, red
facialHairColor: [],
// Possible values: blazer, sweater, shirt, hoodie, overall
clothes: [],
// Possible values: black, blue, gray, heather, pastel, pink, red, white
clothesColor: [],
// Possible values: close, cry, default, dizzy, roll, happy, hearts, side, squint, surprised, wink, winkWacky
eyes: [],
// Possible values: angry, default, flat, raised, sad, unibrow, up
eyebrow: [],
// Possible values: concerned, default, disbelief, eating, grimace, sad, scream, serious, smile, tongue, twinkle, vomit
mouth: [],
Possible values: tanned, yellow, pale, light, brown, darkBrown, black
skin: []
};
jdenticon-sprites 的配置参数:
let options = {
// an array of numbers between 0 and 360
hue: [],
// Distance to the edge of the image
padding: 0,
// an array of numbers between 0 and 1
colorLightness: [],
// an array of numbers between 0 and 1
grayscaleLightness: [],
// an array of numbers between 0 and 1
colorSaturation: [],
// an array of numbers between 0 and 1
grayscaleSaturation: [],
// Any valid color identifier
background: null
};
gridy-sprites 的配置参数:
let options = {
// Use different colors for eyes and mouth
colorful: false
};
initials-sprites 的配置参数:
let options = {
// amber, blue, blueGrey, brown, cyan, deepOrange, deepPurple, agreenmber, grey, indigo, lightBlue, lightGreen, lime, orange, pink, purple, red, teal, yellow
backgroundColors: [],
// background color
// Possible values: 50, 100, 200, 300, 400, 500, 600, 700, 800, 900
backgroundColorLevel: 600,
// font size
// Number between 1 and 100
fontSize: 50,
// Number between 0 and 2
chars: 2,
// bold?
bold: false
};
Github 网址为:https://github.com/DiceBear/avatars
演示地址 | 下载地址 |
专业提供WordPress主题安装、深度汉化、加速优化等各类网站建设服务,详询在线客服!