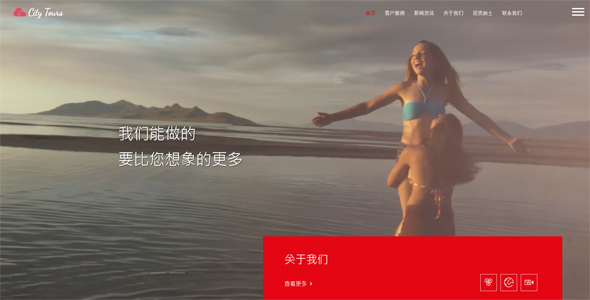
这是一款简单实用的 jQuery 响应式图片无缝轮播插件。该插件会将要轮播的图片制作为背景图片,它支持 ie8+浏览器,具有无限、无缝轮播等特点。
该轮播图插件的基本 HTML 结构如下:
<div class="banner">
<ul>
</ul>
<ol>
</ol>
<i class="left"></i><i class="right"></i>
</div>
使用下面的 CSS 代码来制作轮播图的全屏响应式效果。
.banner {
width: 100%;
position: relative;
overflow: hidden;
}
.banner ul { position: absolute; }
.banner ul li {
width: 100%;
height: 560px;
float: left;
background: no-repeat center center;
}
圆点导航和箭头导航按钮的样式如下:
.banner ol {
height: 20px;
background: rgba(0,0,0,0.5);
position: absolute;
left: 50%;
bottom: 30px;
padding: 0 10px;
border-radius: 10px;
}
.banner ol li {
width: 10px;
height: 10px;
float: left;
margin: 5px 5px;
background: rgba(255,255,255,0.5);
border-radius: 50%;
cursor: pointer;
}
.banner ol .current { background: rgba(255,255,255,1); }
.banner i {
width: 58px;
height: 120px;
position: absolute;
top: 50%;
margin-top: -60px;
cursor: pointer;
border-radius: 5px;
display: none;
}
.banner .left {
left: 60px;
background: url('left_right.png') no-repeat 0 0px;
}
.banner .right {
right: 60px;
background: url('left_right.png') no-repeat 0px -120px;
}
.banner .left:hover, .banner .right:hover { background-color: rgba(0, 0, 0, 0.31); }
在页面中引入 jquery,实现该轮播图效果的 jquery 代码如下:
$(function(){ //页面加载完毕才执行
//=========设置参数==========
//图片统一高度:
var images_height = '560px';
//图片路径/链接(数组形式):
var images_url = [
'img/1.jpg',
'img/2.jpg',
'img/3.jpg'
];
var images_count = images_url.length;
//console.log(images_count);
//创建节点
//图片列表节点
for(var j=0;j<images_count+1;j++){
$('.banner ul').append('<li></li>')
}
//轮播圆点按钮节点
for(var j=0;j<images_count;j++){
if(j==0){
$('.banner ol').append('<li class="current"></li>')
}else{
$('.banner ol').append('<li></li>')
}
}
//载入图片
$('.banner ul li').css('background-image','url('+images_url[0]+')');
$.each(images_url,function(key,value){
$('.banner ul li').eq(key).css('background-image','url('+value+')');
});
$('.banner').css('height',images_height);
$('.banner ul').css('width',(images_count+1)*100+'%');
$('.banner ol').css('width',images_count*20+'px');
$('.banner ol').css('margin-left',-images_count*20*0.5-10+'px');
//=========================
var num = 0;
//获取窗口宽度
var window_width = $(window).width();
$(window).resize(function(){
window_width = $(window).width();
$('.banner ul li').css({width:window_width});
clearInterval(timer);
nextPlay();
timer = setInterval(nextPlay,2000);
});
//console.log(window_width);
$('.banner ul li').width(window_width);
//轮播圆点
$('.banner ol li').mouseover(function(){//用hover的话会有两个事件(鼠标进入和离开)
$(this).addClass('current').siblings().removeClass('current');
//第一张图: 0 * window_width
//第二张图: 1 * window_width
//第三张图: 2 * window_width
//获取当前编号
var i = $(this).index();
//console.log(i);
$('.banner ul').stop().animate({left:-i*window_width},500);
num = i;
});
//自动播放
var timer = null;
function prevPlay(){
num--;
if(num<0){
//悄悄把图片跳到最后一张图(复制页,与第一张图相同),然后做出图片播放动画,left参数是定位而不是移动的长度
$('.banner ul').css({left:-window_width*images_count}).stop().animate({left:-window_width*(images_count-1)},500);
num=images_count-1;
}else{
//console.log(num);
$('.banner ul').stop().animate({left:-num*window_width},500);
}
if(num==images_count-1){
$('.banner ol li').eq(images_count-1).addClass('current').siblings().removeClass('current');
}else{
$('.banner ol li').eq(num).addClass('current').siblings().removeClass('current');
}
}
function nextPlay(){
num++;
if(num>images_count){
//播放到最后一张(复制页)后,悄悄地把图片跳到第一张,因为和第一张相同,所以难以发觉,
$('.banner ul').css({left:0}).stop().animate({left:-window_width},500);
//css({left:0})是直接悄悄改变位置,animate({left:-window_width},500)是做出移动动画
//随后要把指针指向第二张图片,表示已经播放至第二张了。
num=1;
}else{
//在最后面加入一张和第一张相同的图片,如果播放到最后一张,继续往下播,悄悄回到第一张(肉眼看不见),从第一张播放到第二张
//console.log(num);
$('.banner ul').stop().animate({left:-num*window_width},500);
}
if(num==images_count){
$('.banner ol li').eq(0).addClass('current').siblings().removeClass('current');
}else{
$('.banner ol li').eq(num).addClass('current').siblings().removeClass('current');
}
}
timer = setInterval(nextPlay,2000);
//鼠标经过banner,停止定时器,离开则继续播放
$('.banner').mouseenter(function(){
clearInterval(timer);
//左右箭头显示(淡入)
$('.banner i').fadeIn();
}).mouseleave(function(){
timer = setInterval(nextPlay,2000);
//左右箭头隐藏(淡出)
$('.banner i').fadeOut();
});
//播放下一张
$('.banner .right').click(function(){
nextPlay();
});
//返回上一张
$('.banner .left').click(function(){
prevPlay();
});
});
Github 地址:https://github.com/maizhenying09/jquery-seamless-picture-carousel
演示地址 | 下载地址 |
专业提供WordPress主题安装、深度汉化、加速优化等各类网站建设服务,详询在线客服!